MysteriousUser94
Guest
- 0
- Posts
Hello everyone :)
As you can see in this video
I programmed a dynamic lighting system. This system allows you to reproduce things like the Ecruteak City's Gym (in HGSS), it will also allow you to make caves that are a little more alive :)
How to install the script
To install the script, you have to download this file : http://www.mediafire.com/file/utc8164dswlaczb/00500_NuriYuri_DynamicLight.7z/file
And extract it using 7zip into the folder scripts of your project ( ProjectFolder/scripts ).
You will also need the following file : https://www.mediafire.com/file/54zvvn7nl46gxrq/dynamic_light.7z/file to be extracted in graphics/particles.
Start the system
To start the system you must put (in an event) the following script command before the teleportation command :
You can put all your definitions of light before the line end of this command.
For example :
This command will display a zoomable animated light on the hero (which will be off), and animated lights on events 001, 002 and 003. Here's the result :
To understand what happened, here's the detailled explanations.
We told NuriYuri::DynamicLight to execute the indicated instructions between do |dl| and end during the next teleportation. When the instructions are executed, dl contains NuriYuri::DynamicLight (it's an alias). We indicate it to add lights.
The light adding instruction is NuriYuri::DynamicLight.add(event_id, light_type, animation_type, zoom_count, opacity_count, *args)
You can call the function NuriYuri::DynamicLight.start between each teleportation (when the rooms are different). Please note that the script takes care of memorizing your addings and changes in case of the player reloading the game :)
Stop the system
When you leave the cave, it is interesting to disable the DynamicLight System. Nothing complicated about that : add the following command before the teleportation command :
Turn on/off lights
To turn on a light, you can use the following command :
light_index is a number varying between 0 and n-1 where n is the number of .add which you put into the starting command. (0 is the first .add, 1 is the second, etc)
To turn off a light, enter the command :
Detect if a light is on
To know if a light is on, use the following script condition :
Access the zoom of a zoomable light
To access the zoom of a zoomable light, you have to enter the following piece of code :
For example, to know if the hero's light is not big in script condition :
Modify the zoom of a zoomable light
Enter the following command :
Create a new type of zoom animation / opacity
Animations are stored in NuriYuri::DynamicLight::ANIMATIONS, it's an array that contains each animation in the form of a Hash containing the values of the zoom and opacity for each frame of the animation.
To add one you can enter in a new script the following line :
For example, to create the animation 3 displaying a light that is completely haywire :
I invite you to read this part of the Ruby tutorial to understand : Link to the part.
Create a new type of light
Each type of light is stored in the variable NuriYuri::DynamicLight::LIGHTS, this array contains arrays indicating the lights' infos.
The first cell of the info array is the type :
The second cell indicates the file's name in graphics/particles that will serve as the mask to display the light.
The third cell is optional (don't put it if you don't use it). It gives the file's name (in graphics/particles) which will be displayed in the map's viewport.
Example : Add a circle of 128px radius
Code to put in a new script, remember to put the image of the 128px radius circle into graphics/particles/dynamic_light.
Put light_type to 3 to use this new circle of 128px as a mask.
Other infos
This script is available on Gitlab ( https://gitlab.com/NuriYuri/dynamiclightscript ), you can add it and update it using git. You can add this script as a submodule or clone it in the scripts folder :
Or
Note : This script is totally functionnal from PSDK Alpha 24.9 onwards. Before, it lacks a task, in the Scheduler, allowing it to function properly.
___________
Translation by Rey & SMB64.
As you can see in this video
I programmed a dynamic lighting system. This system allows you to reproduce things like the Ecruteak City's Gym (in HGSS), it will also allow you to make caves that are a little more alive :)
How to install the script
To install the script, you have to download this file : http://www.mediafire.com/file/utc8164dswlaczb/00500_NuriYuri_DynamicLight.7z/file
And extract it using 7zip into the folder scripts of your project ( ProjectFolder/scripts ).
You will also need the following file : https://www.mediafire.com/file/54zvvn7nl46gxrq/dynamic_light.7z/file to be extracted in graphics/particles.
Start the system
To start the system you must put (in an event) the following script command before the teleportation command :
Code:
NuriYuri::DynamicLight.start do |dl|
# Light definition
end
You can put all your definitions of light before the line end of this command.
For example :
Code:
NuriYuri::DynamicLight.start do |dl|
dl.add(0, 0, 2, 0, 0, 0.3, type: dl::ScalableDLS)
dl.switch_off(0)
dl.add(1, 2, 2)
dl.add(2, 2, 2)
dl.add(3, 2, 2)
end
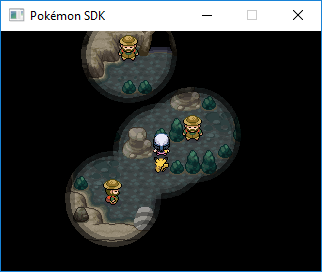
To understand what happened, here's the detailled explanations.
We told NuriYuri::DynamicLight to execute the indicated instructions between do |dl| and end during the next teleportation. When the instructions are executed, dl contains NuriYuri::DynamicLight (it's an alias). We indicate it to add lights.
The light adding instruction is NuriYuri::DynamicLight.add(event_id, light_type, animation_type, zoom_count, opacity_count, *args)
- dl.add(0, 0, 2, 0, 0, 0.3, type: dl::ScalableDLS) adds a zoomable light (it's type: dl::ScalableDLS which indicates it) with a zoom 0.3. Its type is 0 (lights with a circle of 320 px radius) and it's animation is animation n°2 (zoom varying between 0.65 and 0.75, opacity varying between 225 and 255).
- dl.switch_off(0) turns off the light of index 0 (the first one added with dl.add).
- dl.add(1, 2, 2) adds a normal light of type 2 (circle of 96 px radius) and
with animation 2 on the event 001 of the map where we're about to teleport. - dl.add(2, 2, 2) same but with event 002.
- dl.add(3, 2, 2) same but with event 003.
You can call the function NuriYuri::DynamicLight.start between each teleportation (when the rooms are different). Please note that the script takes care of memorizing your addings and changes in case of the player reloading the game :)
Stop the system
When you leave the cave, it is interesting to disable the DynamicLight System. Nothing complicated about that : add the following command before the teleportation command :
Code:
NuriYuri::DynamicLight.stop_delay
Turn on/off lights
To turn on a light, you can use the following command :
Code:
NuriYuri::DynamicLight.switch_on(light_index)
To turn off a light, enter the command :
Code:
NuriYuri::DynamicLight.switch_off(light_index)
Detect if a light is on
To know if a light is on, use the following script condition :
Code:
NuriYuri::DynamicLight.light_sprite(light_index).on
Access the zoom of a zoomable light
To access the zoom of a zoomable light, you have to enter the following piece of code :
Code:
NuriYuri::DynamicLight.light_sprite(light_index).scale
For example, to know if the hero's light is not big in script condition :
Code:
NuriYuri::DynamicLight.light_sprite(0).scale < 0.5
Modify the zoom of a zoomable light
Enter the following command :
Code:
NuriYuri::DynamicLight.light_sprite(0).scale_to(target_zoom, duration)
- target_zoom is the final zoom of the light, for example 0.5
- duration is the duration to go from the current zoom to the final zoom (0 = instant)
Create a new type of zoom animation / opacity
Animations are stored in NuriYuri::DynamicLight::ANIMATIONS, it's an array that contains each animation in the form of a Hash containing the values of the zoom and opacity for each frame of the animation.
To add one you can enter in a new script the following line :
Code:
NuriYuri::DynamicLight::ANIMATIONS[animation_id] = { zoom: [list_zoom], opacity: [list_opacity] }
For example, to create the animation 3 displaying a light that is completely haywire :
Code:
rand_array = Array.new(10) { Math::exp(rand) / 2.71 }
NuriYuri::DynamicLight::ANIMATIONS[3] = {
zoom: Array.new(50) { |i| rand_array[i / 5].to_f },
opacity: [255]
}
Create a new type of light
Each type of light is stored in the variable NuriYuri::DynamicLight::LIGHTS, this array contains arrays indicating the lights' infos.
The first cell of the info array is the type :
- :normal : Doesn't check the direction of its event to change its angle.
- :direction : Check the direction of its event to change its angle.
The second cell indicates the file's name in graphics/particles that will serve as the mask to display the light.
The third cell is optional (don't put it if you don't use it). It gives the file's name (in graphics/particles) which will be displayed in the map's viewport.
Example : Add a circle of 128px radius
Code:
NuriYuri::DynamicLight::LIGHTS[3] = [:normal, 'dynamic_light/circle_128']
Put light_type to 3 to use this new circle of 128px as a mask.
Other infos
This script is available on Gitlab ( https://gitlab.com/NuriYuri/dynamiclightscript ), you can add it and update it using git. You can add this script as a submodule or clone it in the scripts folder :
Code:
git submodule add [email protected]:NuriYuri/dynamiclightscript.git "00500 NuriYuri DynamicLight"
Code:
git clone [email protected]:NuriYuri/dynamiclightscript.git "00500 NuriYuri DynamicLight"
Note : This script is totally functionnal from PSDK Alpha 24.9 onwards. Before, it lacks a task, in the Scheduler, allowing it to function properly.
___________
Translation by Rey & SMB64.