- 68
- Posts
- 3
- Years
- Age 35
- Seen today
Hello,
My name is SpeedyAggron and I have recently started to plan my semi open-world fan game. Before I continued with the details, I wanted to make sure certain aspects needed to work. Those are level scaling based on the number of gym badges and a trainer cards that changes depending on the order of the earned badges. I was successful after some trial and error and I want to share these with this forum. You'll need to edit in several places, but the changes are surprisingly simple. I use the BW Essentials version, so some details can be different.
1. First you have to split up the badges image file in the 'Pictures\Trainer Card' folder. Every gym badge needs to have the same size and a simple file name such as "badgeGrass".
2. We follow with defining an array at the 'Settings' section in the script editor with empty string values that will represent your badges. In my case there will be twenty, so you can change this to the total of badges your region has.
3. Next up is to fill in this array and this happens when you have defeated a gym leader in the second tab of the event. Here you insert the value of the filename of his badge after you added the badge total. You can place them in a cluster of conditional branches based on the order you want to beat the gym leaders. In my example you can choose between the second, third and fourth gym leaders.
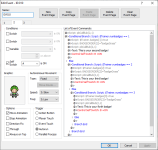
4. The final step is to add this to the trainer card using the array. You simply replace the section where the badges will be displayed with this snippet in the Trainer Card script. This is based on the script to have multiple rows of badges so it will change from your situation. The x and y positions will differ for your trainer card off course and the array will be used to insert all the different badge images.
5. Now that we have our dynamic trainer card, we also need to make the levels of the trainers increase according to the amount of badges we have. For this to work, I have modified this script so the levels won't randomly increase. The levels are pulled from the Trainers.txt file and you can change the level gaps between gym leaders. With this, you won't need clusters of conditional branches and several versions of the same trainer in Trainers.txt. You just need to place the script above 'Main' in the editor and it will automatically change.
I hope this will help people with problems implementing open world fan games. If you find any improvements with if clusters or other aspects, please inform me.
EDIT: I edited step 5 to be more like the original balancer script now that I understand it better. I also forgot to add calcStats in the previous version, so I apologize for people who tried to test that.
My name is SpeedyAggron and I have recently started to plan my semi open-world fan game. Before I continued with the details, I wanted to make sure certain aspects needed to work. Those are level scaling based on the number of gym badges and a trainer cards that changes depending on the order of the earned badges. I was successful after some trial and error and I want to share these with this forum. You'll need to edit in several places, but the changes are surprisingly simple. I use the BW Essentials version, so some details can be different.
1. First you have to split up the badges image file in the 'Pictures\Trainer Card' folder. Every gym badge needs to have the same size and a simple file name such as "badgeGrass".
2. We follow with defining an array at the 'Settings' section in the script editor with empty string values that will represent your badges. In my case there will be twenty, so you can change this to the total of badges your region has.
Code:
#===============================================================================
# * An array that sets the order of the gym badges.
#===============================================================================
BADGEORDER = ["","","","","","","","","","","","","","","","","","","",""]
3. Next up is to fill in this array and this happens when you have defeated a gym leader in the second tab of the event. Here you insert the value of the filename of his badge after you added the badge total. You can place them in a cluster of conditional branches based on the order you want to beat the gym leaders. In my example you can choose between the second, third and fourth gym leaders.
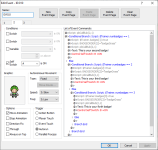
4. The final step is to add this to the trainer card using the array. You simply replace the section where the badges will be displayed with this snippet in the Trainer Card script. This is based on the script to have multiple rows of badges so it will change from your situation. The x and y positions will differ for your trainer card off course and the array will be used to insert all the different badge images.
Code:
x=5
y=605
imagePositions=[]
for j in 0...2
for i in 0...10
if $Trainer.badges[i+j*10]
imagePositions.push(["Graphics/Pictures/TrainerCard/"+BADGEORDER[i+j*10],x,y,0,0,50,50])
end
x+=50
end
x=5
y+=50
end
5. Now that we have our dynamic trainer card, we also need to make the levels of the trainers increase according to the amount of badges we have. For this to work, I have modified this script so the levels won't randomly increase. The levels are pulled from the Trainers.txt file and you can change the level gaps between gym leaders. With this, you won't need clusters of conditional branches and several versions of the same trainer in Trainers.txt. You just need to place the script above 'Main' in the editor and it will automatically change.
Code:
GYME4_TEAM_LIST = [
:LEADER_Cilan,
:LEADER_Cress,
:LEADER_Chili,
]
Events.onTrainerPartyLoad+=proc {|sender,e|
if e[0] # Trainer data should exist to be loaded, but may not exist somehow
trainer=e[0][0] # A PokeBattle_Trainer object of the loaded trainer
items=e[0][1] # An array of the trainer's items they can use
party=e[0][2] # An array of the trainer's Pokémon
# Gym Leader / E4 Battles
ids=GYME4_TEAM_LIST
balance=false
ids.each{|item|balance|=isConst?(trainer.trainertype,PBTrainers,item)
}
if balance
party.each{|poke|
mean = poke.level if $Trainer.numbadges == 0
mean = poke.level+2 if $Trainer.numbadges == 1
mean = poke.level+4 if $Trainer.numbadges == 2
mean = poke.level+6 if $Trainer.numbadges == 3
level=mean
poke.level=level
poke.calcStats
}
end
end
}
I hope this will help people with problems implementing open world fan games. If you find any improvements with if clusters or other aspects, please inform me.
EDIT: I edited step 5 to be more like the original balancer script now that I understand it better. I also forgot to add calcStats in the previous version, so I apologize for people who tried to test that.
Last edited: